I wanted to create a montage from a bunch of images in the same directory. I want to end up with something like the image below. I wanted it to look like a bunch of Polaroids haphazardly arranged on a pinboard.
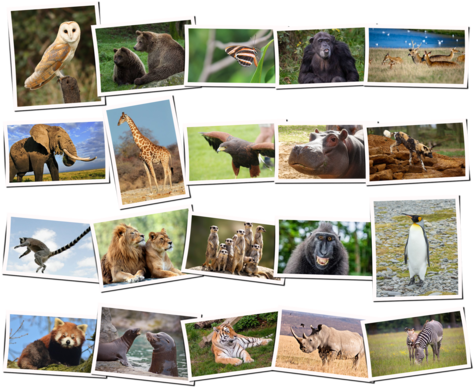
I use bash under Linux. To start with, update and install imagemagick.
sudo apt update
sudo apt install imagemagick
Now create a little script in the same directory as the images I want to use. You can change this script your hearts content.
cat >montage.sh <<EOS
#!/bin/bash
numberofimages=24
center=0
thumbsize=480
offset=400
yoffset=0
count=0
tmpfile=/tmp/montage-tmp.png
outfile=montage-final.png
background=transparent
files=`ls -altr `find . -name 'IMG_*.jpg' -type f -print` | grep -v $outfile | tail -$numberofimages | awk '{ print $9 }'`;
for image in $files
do
# Get image size and determine its virtual canvas
# read, thumbnail, image to a temp file.
convert -size 500x500 "$image" -thumbnail ${thumbsize}x${thumbsize} -bordercolor snow -background black -density 96x96 +polaroid $tmpfile
# Sort out the Y offset to get 6 across and 4 down
if (($count % 6 == 0 )); then yoffset=$(($yoffset+$thumbsize));
center=0;
fi
count=$((count+1));
# location so as to place it's center at the current position.
xpos=`convert "$tmpfile" -format "%[fx: $center - w/2 ]" info:`
ypos=`convert "$tmpfile" -format "%[fx: - h/2 ]" info:`
ypos=$(awk "BEGIN { print $ypos+$yoffset; exit }")
# $xpos and $ypos may be positive, so they may need a '+' sign
xpos=`echo "+$xpos" | sed 's/+-/-/'`
ypos=`echo "+$ypos" | sed 's/+-/-/'`
# Output the image into the pipeline for placement on canvas
# at the position (for top-left corner) calculated
convert -page $xpos$ypos "$tmpfile" MIFF:-
# Increment position for next image
center=`convert xc: -format "%[fx: $center + $offset ]" info:`
done |
# Read the pipeline of the images output from the above loop
# and create the canvas. Repage the virtual canvas, add some
# extra border space and output it.
convert -background "$background" MIFF:- -layers merge +repage -bordercolor "$background" -border 5x5 $outfile
EOS
Now to the script executable and run it.
chmod +x montage.sh
./montage.sh
Without any changes, when you run montage.sh it will grab all the files named IMG_*.jpg in the current directory and generate a montage.
Tweak the various variables at the top of the code to get the image right for you. If your files are named something different, change the ‘IMG_*.jpg’ string to match the names of your files in the files= statement.